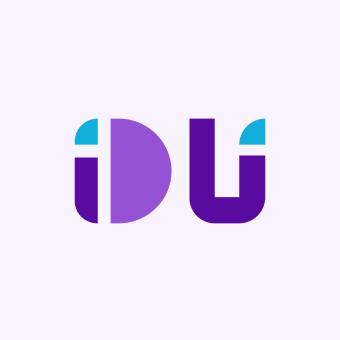
Install
Collapse React Component
Install
npm install --save @idui/react-collapse
yarn add @idui/react-collapse
See props in Docs
Basic Example
import React from 'react'
import Collapse from '@idui/react-collapse'
function Example() {
return <Collapse>
<Collapse.Header>Open</Collapse.Header>
<Collapse.Body>:)</Collapse.Body>
</Collapse>
}
Header Function
import React, { Fragment } from 'react'
import Collapse from '@idui/react-collapse'
function Example() {
return <Collapse>
<Header>
{({ open, close, toggle }) => (
<Fragment>
<button onClick={open}>Open</button>
<button onClick={close}>Close</button>
<button onClick={toggle}>Toggle</button>
</Fragment>
)}
</Header>
<Collapse.Body>:)</Collapse.Body>
</Collapse>
}
Body Function
import React from 'react'
import Collapse from '@idui/react-collapse'
function Example() {
return <Collapse>
<Collapse.Header>Open</Collapse.Header>
<Collapse.Body>
{({ close }) => (
<Button onClick={close}>Close</Button>
)}
</Collapse.Body>
</Collapse>
}
Styling
import React from 'react'
import styled from 'styled-components';
import Collapse from '@idui/react-collapse'
const Header = styled(Collapse.Header)`
padding: 10px;
line-height: 20px;
cursor: pointer;
box-shadow: rgba(0, 0, 0, 0.35) 0 5px 15px;
width: 100%;
`;
const Body = styled(Collapse.Body)`
box-shadow: rgba(0, 0, 0, 0.35) 0 5px 15px;
padding: 20px;
border-bottom-right-radius: 10px;
border-bottom-left-radius: 10px;
width: 100%;
`;
const Content = styled.div`
height: 200px;
display: flex;
align-items: center;
justify-content: center;
`;
function Example() {
return <Collapse>
<Header>Open</Header>
<Body>
<Content>:)</Content>
</Body>
</Collapse>
}
Custom Animation
import React from 'react'
import Collapse from '@idui/react-collapse'
function Example() {
return <Collapse>
<Collapse.Header>Open</Collapse.Header>
<Collapse.Body animation={{
close: {
opacity: 0,
},
open: {
opacity: 1
},
transition: {
open: {duration: 0.2, ease: [0.3, 0.6, 0.3, 0.2]},
close: {duration: 0.1, ease: [0.3, 0.6, 0.3, 0.2]},
},
}}>
:)
</Collapse.Body>
</Collapse>
}
See more details in storybook
License
MIT © [email protected]