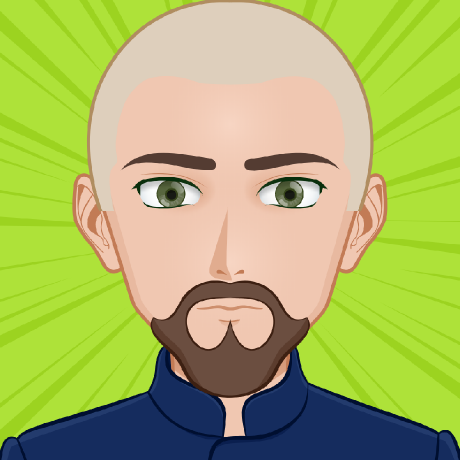
Install
tape-async
A lite wrapper around tape to simplify async testing.
Installation
npm install -D tape-async
Usage
Use with async-await
const test = require('tape-async');
const sleep = require('sleep-promise');
test('this test will successfully pass', async (t) => {
await sleep(100);
const a = await Promise.resolve(42);
t.equal(a, 42);
});
tape-async
supports future ES async-await syntax.
You are in charge to provide traspilation of your test code.
Use with generators
const test = require('tape-async');
const sleep = require('sleep-promise');
test('this test will successfully pass', function *(t) {
const result = yield Promise.resolve(42);
t.equal(result, 42);
});
tape-async
supports generators test to handle async code.
They run using co.
It catch unhandled errors
const test = require('tape-async');
test('this test will fail', () => {
setTimeout(()=>{
throw new Error('unhandled');
}, 100);
});
Unhandled errors in your tests are automatically covered.
Test suite fails with a generic error message and a stack trace.
It catch unhandled Promise
rejections
const test = require('tape-async');
test('this test will fail', () => {
Promise.reject(new Error('unhandled'));
});
Uncatched Promise rejection in your tests are automatically covered.
Test suite fails with a generic error message and a stack trace.
It support every tape features.
const test = require('tape-async');
test.skip('this test will be skipped', () => {
});
test.only('this test will be the only one', t => {
t.equal(42, 42);
t.end();
});
Since this is only a tiny wrapper around tape
, you can
use every feature you are used to.
Related projects
- tape - tap-producing test harness for node and browsers.
License
The MIT License (MIT)
Copyright (c) 2016 Andrea Parodi