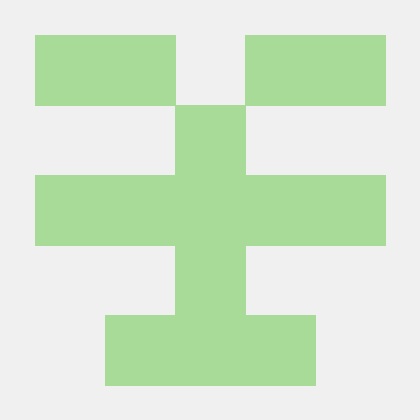
Install
read-glob
Search files with glob pattern and read them, Observable way
const readGlob = require('read-glob');
readGlob('src/*.js').subscribe({
start() {
console.log('Glob started.');
},
next(result) {
result.cwd; //=> '/Users/shinnn/exmaple'
result.path; //=> 'src/a.js'
result.contents; //=> <Buffer ... >
},
complete() {
console.log('Glob completed.');
}
});
Installation
npm install read-glob
API
const readGlob = require('read-glob');
readGlob(pattern [, options])
pattern: string
(glob pattern)
options: Object
(node-glob
and fs.readFile
options) or string
(encoding)
Return: Observable
(zenparsing's implementation)
When the Observable
is subscribed, it starts to search files matching the given glob pattern, read their contents and successively send results to its Observer
.
Results
Each result is the same Object
as glob-observable
's with the additional contents
property, a Buffer
or string
of the matched file contents.
contents
is a string
when the encoding
option is specified, otherwise it's a Buffer
.
readGlob('hi.txt').subscribe(result => {
result.contents; //=> <Buffer 48 69>
});
readGlob('hi.txt', 'utf8').subscribe(result => {
result.contents; //=> 'Hi'
});
readGlob('hi.txt', 'base64').subscribe(result => {
result.contents; //=> 'SGk='
});
options
The option object will be directly passed to node-glob
and fs.readFile
, or the encoding string sets the encoding of fs.readFile
.
Unlike the original node-glob API,
silent
andstrict
options aretrue
by default.nodir
andmark
options are not supported as it ignores directories by default.
const readGlob = require('read-glob');
// ./directory/.dot.txt: 'Hello'
readGlob('*.txt', {
cwd: 'directory',
dot: true
}).subscribe(({contents}) => {
contents.toString(); //=> 'Hello'
});
Related project
- read-glob-promise (Promise version)
License
ISC License © 2017 - 2018 Shinnosuke Watanabe