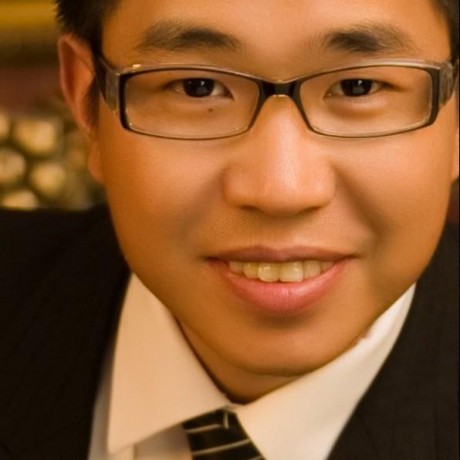
Install
esm-utils
Utilities you'll need when migrating to ESModule.
Install
yarn add esm-utils
Usage
import createEsmUtils from 'esm-utils'
const {
dirname,
filename,
require,
importModule,
resolve,
readJson,
readJsonSync,
} = createEsmUtils(import.meta)
/* Those named exports only accept absolute path or URL */
import {
importModule,
readJson,
loadJson,
readJsonSync,
loadJsonSync,
} from 'esm-utils'
API
createEsmUtils(import.meta | URL | 'string')
Returns an object
with the following properties:
dirname
(alias__dirname
)filename
(alias__filename
)require
importModule
(aliasimport
)resolve
readJson
(aliasloadJson
)readJsonSync
(aliasloadJsonSync
)
Please read this note before you use dirname
and filename
Sync version of readJson
.
utils.importModule(string | URL, options?)
Same as import()
, but accepts absolute path (on Windows, import('C:\\foo.js')
error throws when pass a absolute path starts with a drive letter).
options.traceSyntaxError
type: boolean
default: false
Due to this Node.js issue, Node.js does not emit the location of the syntax error in the error thrown in dynamic import()
.
When set traceSyntaxError: true
, we'll try to get a better error message by running node <file>
in a child process.
utils.readJson(string | URL)
Returns Promise<jsonObject>
.
utils.readJsonSync(string | URL)
Sync version of utils.readJson
utils.resolve(string | URL)
Ponyfill for import.meta.resolve
.
If import.meta.resolve
exits, use it directly, otherwise returns a simple wrapper of import-meta-resolve.
Import json file
With Import Assertions
import foo from './foo.json' assert {type: 'json'}
await import('./foo.json', {assert: {type: 'json'}})
With require
, like CommonJS
import createEsmUtils from 'esm-utils'
const {require} = createEsmUtils(import.meta)
const foo = require('./foo.json')
With readJson
or readJsonSync
import createEsmUtils from 'esm-utils'
const {readJson} = createEsmUtils(import.meta)
const foo = await readJson('./foo.json')
import createEsmUtils from 'esm-utils'
const {readJsonSync} = createEsmUtils(import.meta)
const foo = readJsonSync('./foo.json')
importModule()
Same as utils.importModule()
, but only accept absolute path or URL.
readJson()
(alias loadJson
)
Same as utils.readJson()
, but only accept absolute path or URL.
readJsonSync()
(alias loadJsonSync
)
Same as utils.readJsonSync()
, but only accept absolute path or URL.
You don't need dirname
and filename
The dirname
and filename
supposed to be a quick solution when migrating to ES Modules. In most cases, you don't need them, because many APIs accept URL
directly.
/* BAD */
import fs from 'node:fs/promises'
import path from 'node:path'
import createEsmUtils from 'esm-utils'
const {dirname} = createEsmUtils(import.meta)
const buffer = await fs.readFile(
path.join(dirname, './path/to/file')
)
/* GOOD */
import fs from 'node:fs/promises'
const buffer = await fs.readFile(
new URL('./path/to/file', import.meta.url)
)